Exploring AI PHP and HTML for Web Development
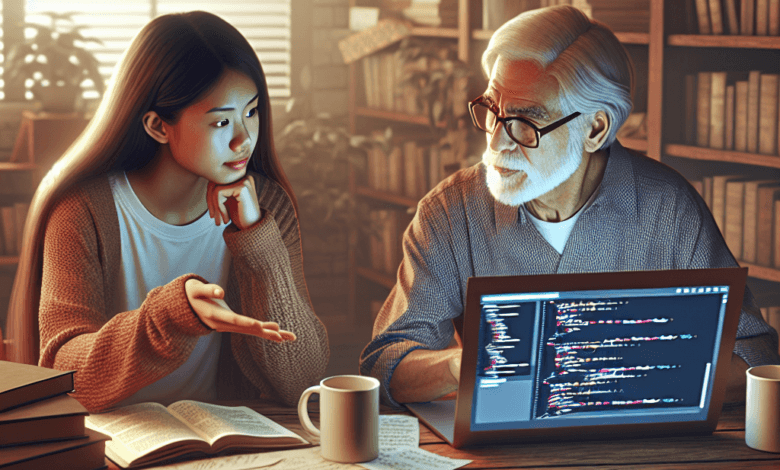
AI PHP and HTML are two fundamental languages used for web development. PHP is a server-side scripting language that is used to create dynamic web pages, while HTML is a markup language used to create the structure and content of web pages. In this article, we will discuss how to write PHP and HTML code with the AI Coder, and provide examples of both languages in action like an AI HTML form.
Writing AI PHP code
PHP code is embedded within HTML code using special delimiters <?php and ?>. This allows PHP to be executed on the server before the web page is sent to the client’s browser. Here is an example of a simple PHP script that displays “Hello, World!” on a web page:
<!DOCTYPE html>
<html>
<body>
<?php
echo "Hello, World!";
?>
</body>
</html>
In this example, the PHP code <?php echo “Hello, World!”; ?> is embedded within the HTML code. When the web page is loaded, the PHP code is executed on the server and the output is sent to the client’s browser.
PHP code can also be used to interact with databases, process form data, and perform other server-side tasks. So here is an example of a PHP script that connects to a MySQL database and displays the results of a query:
<!DOCTYPE html>
<html>
<body>
<?php
$servername = "localhost";
$username = "username";
$password = "password";
$dbname = "database";
// Create a connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check the connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// Perform a query
$sql = "SELECT * FROM table";
$result = $conn->query($sql);
// Display the results
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
echo "Name: " . $row["name"] . " - Age: " . $row["age"] . "<br>";
}
} else {
echo "0 results";
}
// Close the connection
$conn->close();
?>
</body>
</html>
In this example, the PHP code connects to a MySQL database using the mysqli class, performs a query to select all rows from a table, and displays the results on the web page.
Writing AI HTML code
HTML is used to create the structure and content of web pages. It consists of tags that define the elements of a web page, such as headings, paragraphs, links, and images. So here is another example of a simple HTML page that displays a heading and a paragraph of text:
<!DOCTYPE html>
<html>
<body>
<h1>This is a heading</h1>
<p>This is a paragraph.</p>
</body>
</html>
In this example, the HTML tags <h1> and <p> define a heading and a paragraph, respectively. When the web page is loaded, the browser renders these elements according to the specified tags.
HTML can also be used to create forms for user input. Also, here is an example of an AI HTML form that allows users to enter their name and submit it to a PHP script:
<!DOCTYPE html>
<html>
<body>
<form action="process.php" method="post">
Name: <input type="text" name="name">
<input type="submit" value="Submit">
</form>
</body>
</html>
So in this example, the AI HTML form element <form> defines a form that submits data to a PHP script named “process.php” using the HTTP POST method. The form contains an input field for the user’s name and a submit button.
Combining PHP and HTML
However, remember PHP and HTML can be combined to create dynamic web pages that respond to user input and interact with databases. So here is an example of a PHP script that processes form data and displays a personalized greeting on a web page:
<!DOCTYPE html>
<html>
<body>
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$name = $_POST["name"];
echo "Hello, $name!";
}
?>
<form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post">
Name: <input type="text" name="name">
<input type="submit" value="Submit">
</form>
</body>
</html>
So, in this example, the PHP code checks if the form has been submitted using the $_SERVER array and processes the submitted data using the $_POST array. If the form has been submitted, the script displays a personalized greeting with the user’s name.
Conclusion
To conclude, in this article, we have discussed how to write PHP and HTML code with the help of AI Tools, and provided examples of both languages in action. PHP is a server-side scripting language that is used to create dynamic web pages, while HTML is a markup language used to create the structure and content of web pages. By combining PHP and HTML, developers can create interactive web pages that respond to user input, interact with databases, and perform other server-side tasks.